Resolving Unsigned APK Issue in Android Build
Encountered an issue with unsigned APKs? Our investigation revealed missing signingConfig in build.gradle.kts. By setting signingConfig directly within each product flavor, you can ensure your APKs are properly signed. Read on to see the solution and fix your build issues.
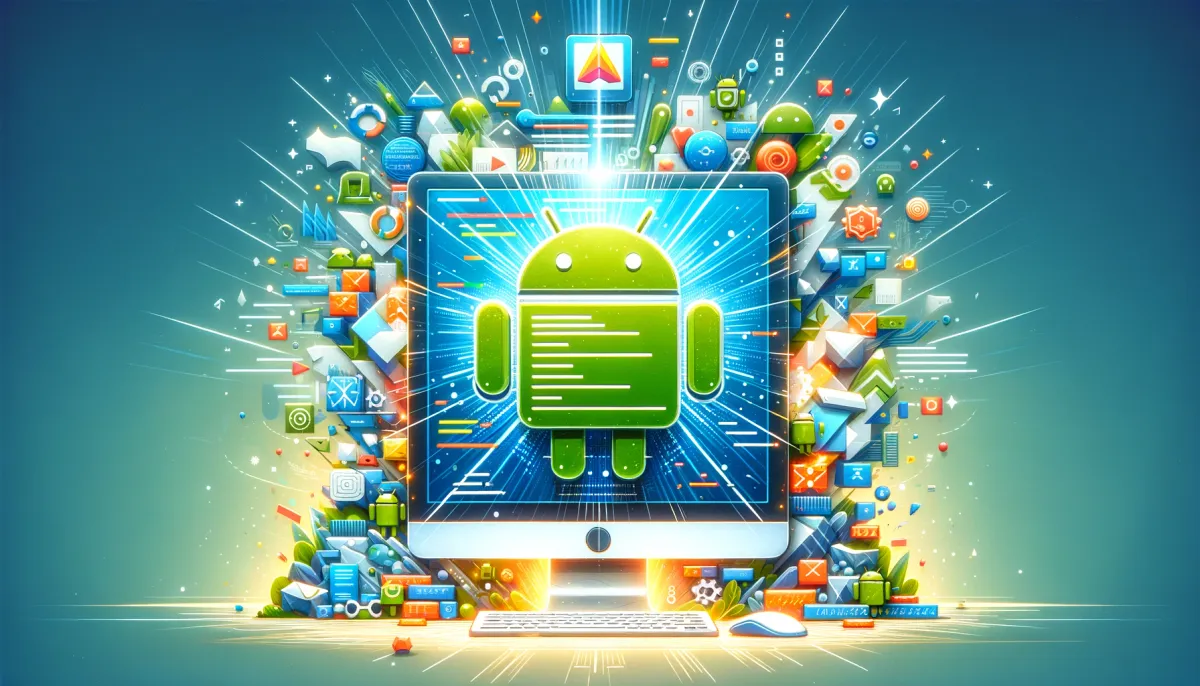
Recently, we encountered an issue where the generated APK had no signing information. When using the following command:
keytool --printcert --jarfile <apk_file_path>
The output indicated that the APK was not a signed JAR file:
Not a signed jar file
Investigation
Upon investigating the build.gradle.kts
file in our project, I found the following settings for the signingConfig
:
productFlavors {
create("app1") {
dimension = "app"
applicationId = "com.example.app1"
versionCode = 1
versionName = "1.0"
createSigningConfig(name, "app1.properties")
}
create("app2") {
isDefault = true
dimension = "app"
applicationId = "com.example.app2"
versionCode = 1
versionName = "1.0"
createSigningConfig(name, "app2.properties")
}
}
Solution
To ensure the APK is signed, set the signingConfig
directly within each product flavor. The updated configuration should look like this:
productFlavors {
create("app1") {
dimension = "app"
applicationId = "com.example.app1"
versionCode = 1
versionName = "1.0"
signingConfig = createSigningConfig(name, "app1.properties")
}
create("app2") {
isDefault = true
dimension = "app"
applicationId = "com.example.app2"
versionCode = 1
versionName = "1.0"
signingConfig = createSigningConfig(name, "app2.properties")
}
}
By setting the signingConfig
on the product flavor, it will be used when building the application, ensuring that the APK is properly signed.