Crafting Custom Build Tasks for Multi-Dimensional Android Builds
In our project, we have defined two dimensions: application (app) and store. For the app dimension, we have two instances: ‘app1’ and…
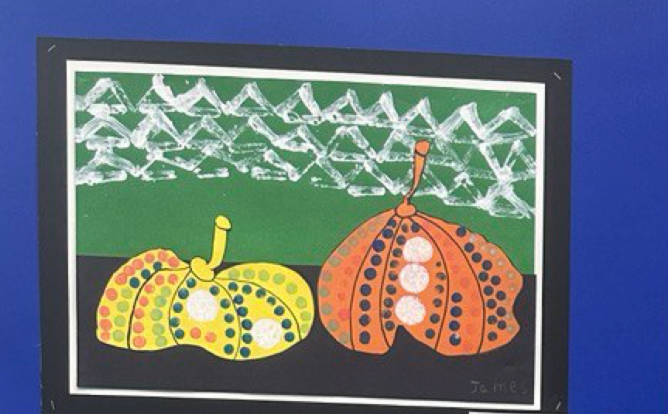
In our project, we have defined two dimensions: application (app) and store. For the app dimension, we have two instances: ‘app1’ and ‘app2’. For the store dimension, we have ‘GooglePlay’ and ‘Website’. In addition to these, we also have two build types: ‘debug’ and ‘release’.
Given these settings, we have tasks such as ‘assembleApp1GooglePlayDebug’ and ‘assembleApp1GooglePlayRelease’, as well as ‘assembleApp1Debug’ and ‘assembleApp1Release’. These tasks allow us to assemble different versions of our app for different platforms and build types.
However, there might be situations where we need to build for ‘app1’ across both ‘GooglePlay’ and ‘Website’ stores, but only for the ‘release’ version. Unfortunately, there isn’t a predefined task for this. The good news is that we can create a custom task to handle this scenario. Here’s how:
First, we define our store and app flavors:
val storeFlavors = listOf("GooglePlay", "Website")
val appFlavors = listOf("app1", "app2")
Next, we create a function to generate our custom build task:
fun createBuildTask(appFlavor: String, buildType: String) {
val taskName = "assemble${appFlavor.capitalize()}${buildType.capitalize()}"
tasks.register(taskName) {
storeFlavors.forEach { storeFlavor ->
dependsOn("assemble${appFlavor.capitalize()}${storeFlavor.capitalize()}${buildType.capitalize()}")
}
}
}
Finally, for each app flavor, we call our function to create the ‘release’ build task:
appFlavors.forEach { appFlavor ->
createBuildTask(appFlavor, "release")
}
With this setup, we can now use the following command to build both the ‘app1GooglePlayRelease’ and ‘app1WebsiteRelease’ versions:
./gradlew assembleApp1Release
This command will trigger the build process for ‘app1’ across both ‘GooglePlay’ and ‘Website’ stores, but only for the ‘release’ version.