Create file structure according to the tree result
Generate structure without data with tree and python script.
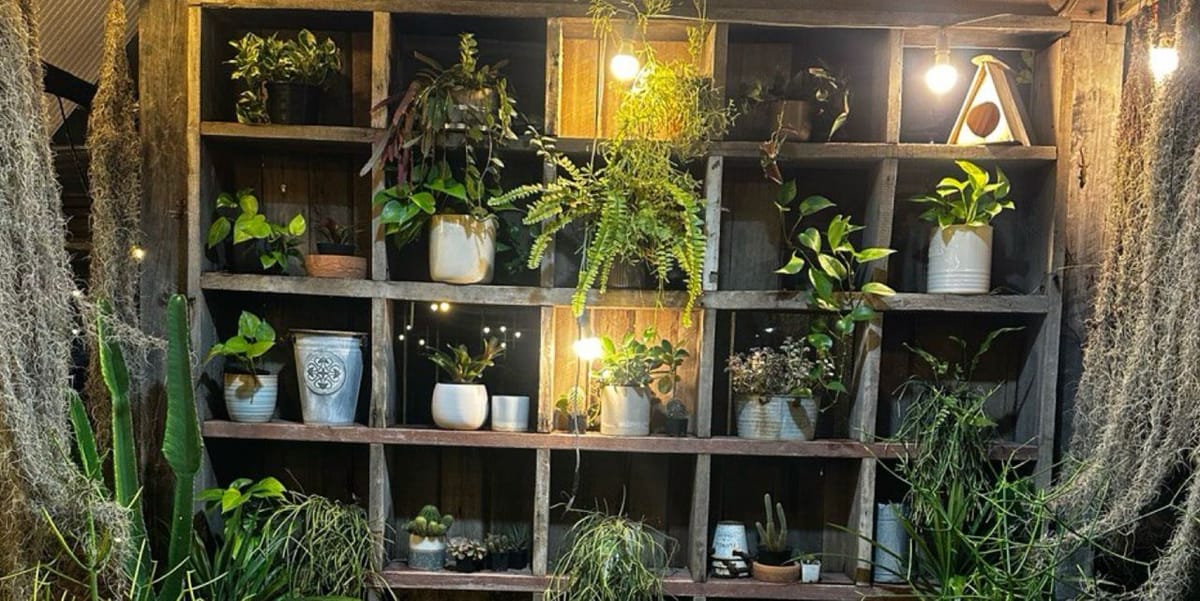
I have a directory in remote machine with large files, and I want to create the file structure the same as in the remote file structure. There was a tree
command, we can use tree -J
command to get the file structure in json format, so I think that we can create the file structure according to the tree -J
result.
Get the file structure with tree command
tree -J <root dir> > root_dir_tree.json
# copy the root_dir_tree.json to local machine
Create file structure with python
Create a file named with create_struture.py
#!/usr/bin/env python3
import json
import os
import sys
def create_structure(contents, parent_path='.'):
for item in contents:
# Skip the report object
if 'name' not in item:
continue
name = item['name']
path = os.path.join(parent_path, name)
if item['type'] == 'directory':
os.makedirs(path, exist_ok=True)
create_structure(item['contents'], path)
elif item['type'] == 'file':
open(path, 'a').close()
def main(json_file_path):
# Load the JSON structure from the file provided as an argument
with open(json_file_path, 'r') as f:
tree = json.load(f)
# Create the directory structure
create_structure(tree)
if __name__ == '__main__':
if len(sys.argv) != 2:
print("Usage: python create_structure.py <path_to_json_file>")
sys.exit(1)
json_file_path = sys.argv[1]
main(json_file_path)
Use the command to create the structure
create_structure.py root_dir_tree.json