Dart: null safety variable and function
null safety variable
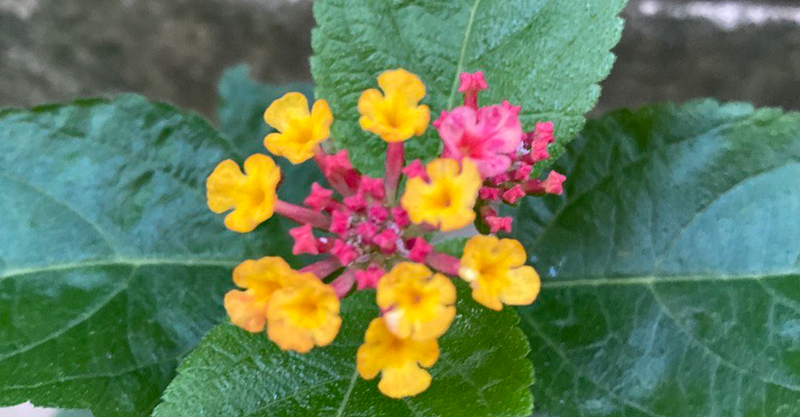
null safety variable
In dart, we can check whether a property is null or not, and inside the if check we can get the value directly, for example:void printValue(int? value) {
if (value != null) {
if (kDebugMode) {
print(1 + value);
}
}
}
But if we define the value in the Widget or on the State object, then we can’t check the null like below:if (widget.value != null) {
if (kDebugMode) {
print(1 + widget.value);
}
}
But we can do like below:int? value = widget.value;
if (value != null) {
if (kDebugMode) {
print(1 + value);
}
}
From the code above, the first example that the we can use the value inside the if check because the value is a parameter, and it can’t be changed, but for the Widget or State, it is a member field, and it can be changed, so we can’t get the value inside the if check.
null safety function
If I define a function on the widget, then how can I call the method? We can use ?.call
to call the function, for example, I define an onDelete
function like below:final Function(int)? onDelete;
We can call the onDelete
function like below:widget.onDelete?.call(1);