Get image base64 string before uploading file
I was trying to get the image’s base64 string without upload files to server, and find that we can use FileReader.readAsDataURL to…
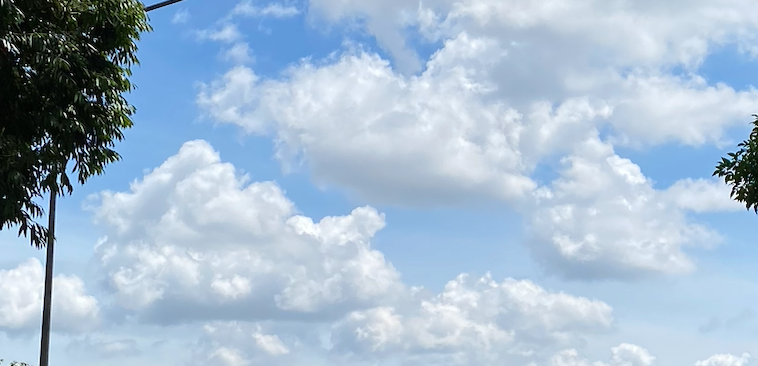
I was trying to get the image’s base64 string without upload files to server, and find that we can use FileReader.readAsDataURL
to implement it.
I wrote a demo page on codepen to show how to get the base64 data of images, the codes to handle the file are like below:var imgContainer = document.getElementById('imgContainer');function handleFiles(input) {
const files = input.files;
for (let i = 0; i < files.length; i++) {
var reader = new FileReader();
reader.onload = function () {
var imgElement = document.createElement('img');
imgElement.setAttribute('src', this.result);
imgContainer.appendChild(imgElement);
};
reader.readAsDataURL(files[i]);
}
}
The main code for getting the base64 string of the file is readAsDataURL
function, before we call this function, we set the callback of the onload
, when FileReader
finished to read the content, it will trigger onload
event, and we can get the base64 string from the result.
There are some more examples for how to get the base64 string on readAsDataURL
document.
Resources