How to connect a domain with specific ip address
Sometimes, we might configure multiple ip address to a domain, or sometimes the domain does not work in some places, so I want to know how…
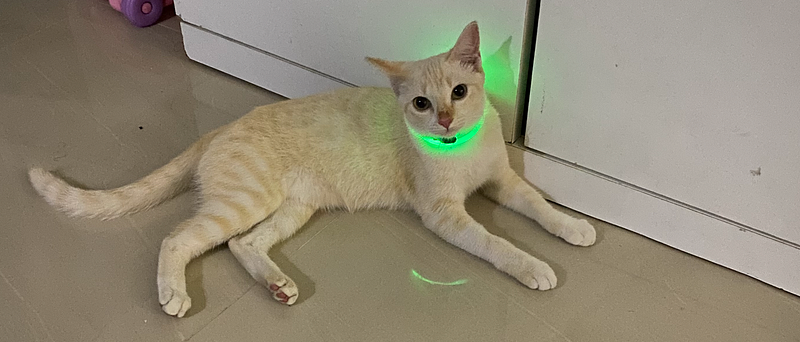
Sometimes, we might configure multiple ip address to a domain, or sometimes the domain does not work in some places, so I want to know how to specify the ip address when connect to the domain.
Specify ip address when connect with curl
I want to connect to a domain with specify ip address, and I find that we can use the --resolve
to do it, I get the help from How to test a HTTPS URL with a given IP address. The command is like below:
curl https://www.google.com --resolve 'www.google.com:443:216.58.199.228'
Implement it in golang
Then I want to know how to implement it in golang, I find the help from golang force http request to specific ip (similar to curl — resolve), the following code is an example.
func main() {
dialer := &net.Dialer{
Timeout: 30 * time.Second,
KeepAlive: 30 * time.Second,
// DualStack: true, // this is deprecated as of go 1.16
}
// or create your own transport, there's an example on godoc.
http.DefaultTransport.(*http.Transport).DialContext = func(ctx context.Context, network, addr string) (net.Conn, error) {
if addr == "www.google.com:443" {
addr = "216.58.199.228:443"
}
return dialer.DialContext(ctx, network, addr)
}
resp, err := http.Get("https://www.google.com")
log.Println(resp.Header, err)
content, err := ioutil.ReadAll(resp.Body)
if err != nil {
fmt.Printf("Failed to read content, the error is %v", err)
}
fmt.Printf("The body is %s\n", string(content))
}