How to fetch a variable value which name is from another variable in bash
Problem
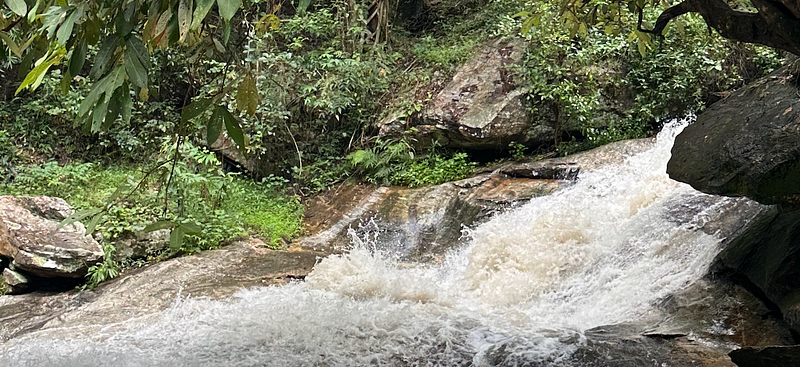
Problem
I want to implement a function like this, I have variables like below, and I want to print the value of ${app}_title, for example, when the app is app1, it will print Application 1, if app is app2, then it will print Application 2.
app1_title="Application 1"
app2_title="Application 2"
app="app1"
Solution
The implementation is like below:
app_title="${app}_title"
echo ${!app_title}
In this script, app_title
is a variable that holds the string "app1_title". The ${!app_title}
syntax is used to get the value of the variable whose name is stored in app_title
, which is "Application 1". This feature of bash is called variable indirection.
Full Example
app1_title="Application 1"
app2_title="Application 2"
app="app1"
if [ ! -z $1 ]; then
app="$1"
fi
app_title_var=${app}_title
echo "The app title is ${!app_title_var}"
If the bash file is called var.sh
, then the output is like below:
$ ./var.sh
The app title is Application 1
$ ./var.sh app2
The app title is Application 2