Passing Parameters to Tasks in Makefile
In this installment of our Makefile series, we explore how to pass parameters to tasks, using the example of downloading JSON playlists from YouTube with youtube-dl. Learn how to enhance your Makefile to accept dynamic parameters, making your tasks more flexible and efficient.
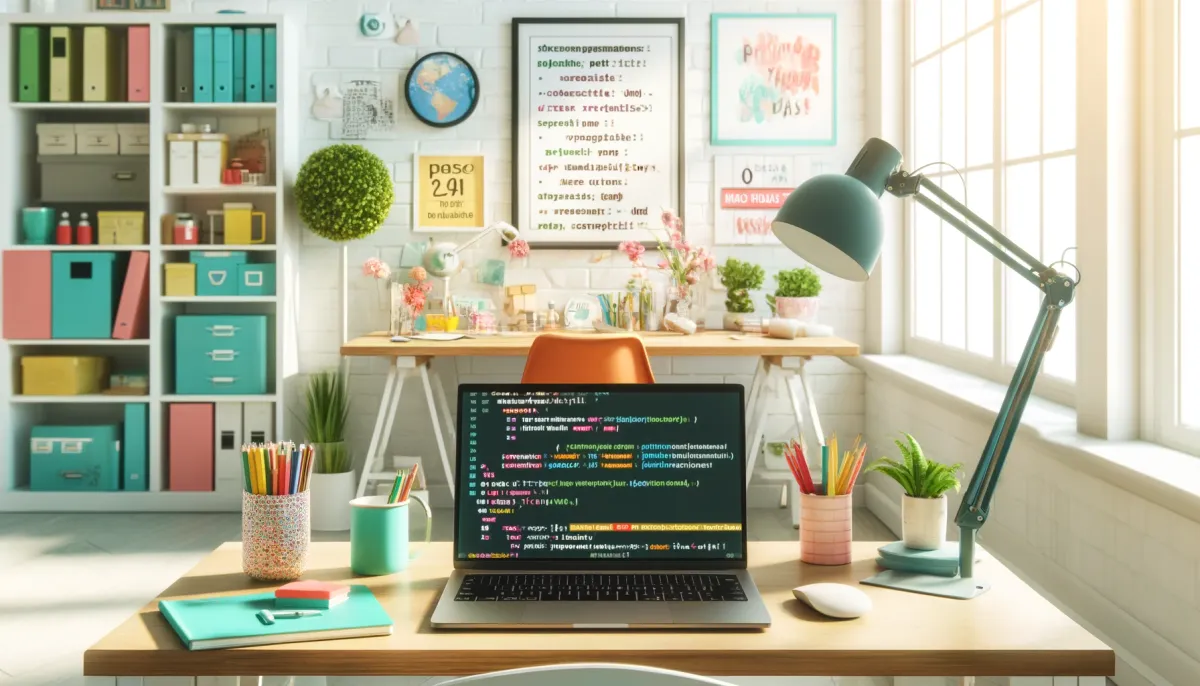
In this article, part of our ongoing Makefile series, I will demonstrate how to pass parameters to tasks in a Makefile.
Example
Consider the useful tool youtube-dl
, which is used to download videos from YouTube. It also has a flag to download the JSON list of a playlist. The command looks like this:
youtube-dl -J https://www.youtube.com/playlist?list=<playlist_id>
I want to create a Makefile task to download the JSON file simply, as shown below:
download-youtube-playlist-json:
@youtube-dl -J "https://www.youtube.com/playlist?list=<playlist_id>" > <playlist_id>.json
In this example, the playlist_id
is hardcoded in the Makefile. If I want to download another JSON list, I need to update the Makefile.
Solution
To simplify the task, we can update the Makefile to accept a parameter, allowing us to download different playlist JSON files with the same task but different parameters. The modified Makefile is shown below:
PLAYLIST_ID=$(list)
download-youtube-playlist-json:
ifeq ($(strip $(PLAYLIST_ID)),)
$(error list is empty. Please run the task like: make download-youtube-playlist-json list=<playlist_id>)
endif
@youtube-dl -J "https://www.youtube.com/playlist?list=$(PLAYLIST_ID)" > $(PLAYLIST_ID).json
Explanation
Variable Definition
PLAYLIST_ID=$(list)
- This line defines a variable
PLAYLIST_ID
which is assigned the value of another variablelist
. When you run themake
command, you can pass a value tolist
withlist=<playlist_id>
.
Conditional Check
ifeq ($(strip $(PLAYLIST_ID)),)
$(error list is empty. Please run the task like: make download-youtube-playlist-json list=<playlist_id>)
endif
- The
ifeq ($(strip $(PLAYLIST_ID)),)
directive checks ifPLAYLIST_ID
is empty after stripping any leading or trailing whitespace. - If
PLAYLIST_ID
is empty, the$(error ...)
function is invoked, which stops the make process and prints the error message: "list is empty. Please run the task like: make download-youtube-playlist-json list=<playlist_id>". - Note that the directive should not be indented.
Usage
To use the task to download the JSON of a YouTube playlist, you can use the following command:
make download-youtube-playlist-json list=<playlist_id>
By using this approach, you can easily pass different playlist IDs to the Makefile task without modifying the Makefile itself each time. This makes the process more efficient and flexible.