The git commands that I use frequently
I list the git commands I use frequently, some of them I will search the internet when using, so I save them here, and next time I can…
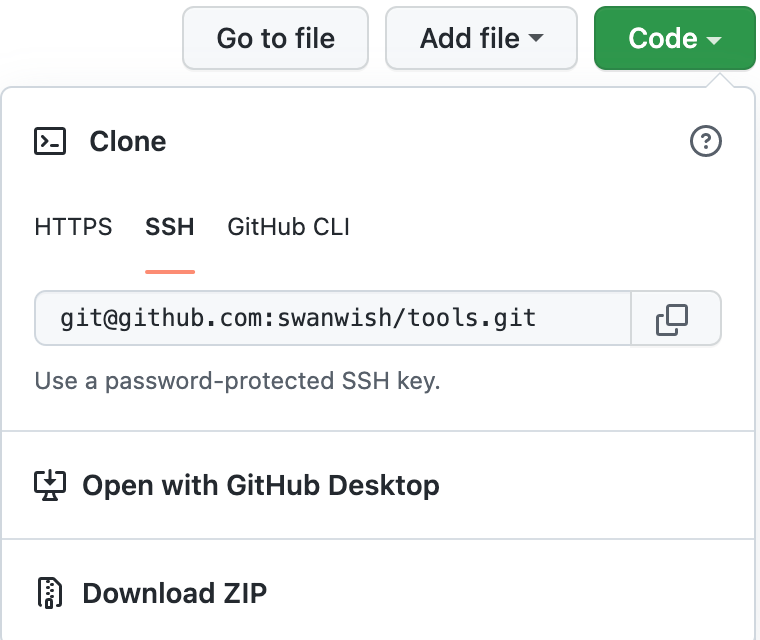
I list the git commands I use frequently, some of them I will search the internet when using, so I save them here, and next time I can find them here quickly.
Git is a distributed version control system, it is used a lot nowadays, we also use it on our daily work. The following commands is what I used frequently in my work.
git init
Init git in one directory, use the terminal to the directory, then run the git init
command.git init
git clone
Get code from git repository, first we need to get the git remote url, then use the git clone
command to get the files. For example, when click the code button on one of my repository, the git url will shown on a popup dialog as below:
To get the code on the repository, then we can use the clone command like below:# git protocol
git clone git@github.com:swanwish/tools.git# https protocol
git clone https://github.com/swanwish/tools.git
git add
git add
is used to add files to stage area, after add the file, the git commit
command will save the changes to git.# Add all files on current directory
git add .# Add one file
git add <filepath>
# for example
git add test/test1.txt# Add all files inside a folder
git add <folderpath>
# for example, if there is a folder named test in current directory
git add test
git commit
git commit
will added the changes to git.# Commit with a comment, the message can be multiple lines
git commit -m 'comment message'# Just commit, and input the message in vi
git commit
Modify last commit
With the following command, we can add changes to last commit, we can change the last commit message, and the changes will be included together with last commit.git commit --amend
git reset
git reset
can be used to undo changes, for example, when I use git commit
add the changes to a wrong branch, when I can use the command to undo changes. git reset has two types, one is hard, and the other is soft.
- hard reset will delete the change and reset to the place you specified.
- soft reset will keep the changes in local workspace.
I usually when I commit to wrong branch, or I forget update the codebase before commit the changes, then I will reset the commit, and stash the local changes, update the codebase, then pop the stash and commit the changes.
soft reset# Soft reset, with one commit
git reset --soft HEAD~1
# If you want to undo 5 commits, then change 1 to 5
git reset --soft HEAD~5
hard resetgit reset --hard HEAD~1
git branch
When we develop a large project, or develop new feature, when create a branch to work is a better idea. The branch contain local branch and remote branch. When we create a branch, it only exists in local workspace, we need to push the branch to the remote, then it will exists in remote.
Create a local branch
The following command will create a branch with current state, for example, you can checkout a commit, and create a branch on that commit.git checkout -b <branch_name>
Push branch to remotegit push -u origin <branch_name>
Rename local branchgit branch -m <new_branch_name>
Rename remote branch# Before use the following command, the local branch name should be updated
# The command below is delete the old branch name and create a new branch
git push origin :<old_branch_name> <new_branch_name># The following command is used to update local upstream branch
git push -u origin <new_branch_name>
Delete local branchgit branch -d <branch_name># Force deleting a branch
git branch -D <branch_name>
Delete remote branch
To delete the remote branch, we need to use git push
command like below:git push --delete <remote_name> <branch_name># example
git push --delete origin test_branch
List branches# List local branches
git branch# List remote branches
git branch -r# List all branches
git branch -a
git tag
Git supports two types of tags: lightweight and annotated.
A lightweight tag is very much like a branch that doesn’t change — it’s just a pointer to a specific commit.
Annotated tags, however, are stored as full objects in the Git database.
Create local tag# Annotated tags
git tag -a <tag_name> -m "<tag message>"# Lightweight tags
git tag <tag_name># Create tag on a specific commit with commit id
git tag -a <tag_name> <commit_id>
git tag <tag_name> <commit_id>
Push tags to remote# Push one tag, just like the branch
git push origin <tag_name># Push all local tags
git push --tags
Delete tags# Delete local tag
git tag -d <tag_name># Delete remote tag
git push <remote> :refs/tags/<tag_name>
git push <remote> --delete <tag_name># Example
# delete local tag (v1.0)
git tag -d v1.0
# delete remote tag (v1.0)
git push origin :refs/tags/v1.0
git push origin --delete v1.0
List tagsgit tag
git tag -l
git tag --list# Search tags
git tag -l "v1*"
git stash
When we change some files on one branch, and if we want to move to another branch to change files, but we don’t want to discard the changes or commit the changes, then we can use stash.# Stash current changes
git stash# List stashes
git stash list
--keep-index option
This tells Git to not include all staged content in the stash being created, but simultaneously leave it in the index.git stash --keep-index
--include-untracked
or -u
option
This tells Git to include untracked files in the stash being created. However, the files that was explicitly ignored will not included. To include ignored files, use --all
or -a
.git stash -u
git stash --include-untracked
Apply changes# Apply the changes and drop the stash
git stash pop# Apply stash changes
# apply the most recent stash
git stash apply# apply a specify stash by name, for example by name stash@{2}
git stash apply stash@{2}
git clean
Clean local workspace, we can use git clean
command.git clean# To remove all the untracked files in your working directory
git clean -f -d# --dry-run (-n) option
git clean -n -d# git clean won't include files are ignored in the .gitignored, in order to clean these files, we can use -x option
git clean -n -d -x# interactive mode -i option
git clean -x -i
git checkout
We can use git checkout
command to checkout the code, we can navigate to a specify commit by provide the commit id, or checkout a branch by provide the branch name, or checkout a tag with the tag name.git checkout <commit_id>
git checkout <branch_name>
git checkout <tag_name># Create a branch
git checkout -b <branch_name># Discard changes
git checkout -- <file or directory># Discard all changes from current directory
git checkout -- .
git rebase
We use develop branch to develop, and create a branch for current change, for example, my current branch is feature_220504, if this branch is changed for a long time, and the develop branch already has a lot of changes, then I can use rebase command to include the changes in develop branch to my current branch with the following command.# Change to develop branch
git checkout develop# Get changes on the develop branch
git pull# Change back to my branch
git checkout feature_220504# Rebase with develop branch
git rebase develop
If the code has conflict, then it will stop to let we merge the codes, after merge the codes, then we can use git rebase --continue
to continue the rebase procedure, or use git rebase --abort
to abort the rebase.# Continue the rebase
git rebase --continue# Abort the rebase
git rebase --abort
git merge
Merge the changes from one branch to another branch. The command will create a new commit.git merge <branch_name># For example, merge develop to branch_220504
git checkout branch_220504
git merge develop
git restore
If we added the changed files to the git with git add
command, then we can use git restore to move it back.git restore --staged <file>...
git log
With git log
command, we can visit all logs of the git repository, there are some options we can use with the git log
command.
Oneline
The --oneline
option will print each commit to a single line.git log --oneline
git status
Another command I used most is git status
command, this output will show some helps, for example git restore
or git checkout
git status